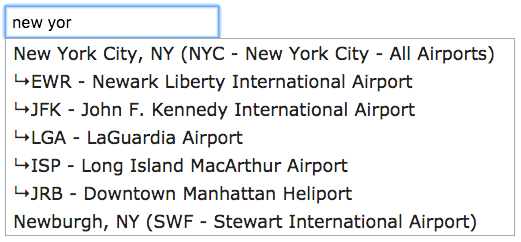
This is your best solution if you need to build an airport autocomplete field like what you experience in the input field at the top of this page.
The endpoint returns all of the airports matching your search term and sorts them based on airport size and servicing area. It will even nest airports within any of the 37 grouped municipal airport codes which show up in common searches like New York, Chicago, London, etc.
For every keypress, you make a request and we send back airport information (in a json string) for related airports matching your input field value.
You can use any popular front-end code to manage the display of the data. We use JQueryUI's autocomplete functionality for our demos, but any will work.
Download our example jQuery code, it comes with JavaScript the Air-port-codes javascript library and some use case examples that make connecting to our API a breeze. Your API key can be found in your air-port-codes.com account and is necessary to be granted access privileges to any of our API's.
Use our interactive REST tool (Swagger) or our Postman collection to help you test the api and build your queries the right way. You'll need to login before you can use it with your API key.
Quick start
First login to your air-port-codes.com account and update your account settings by adding any domains that might need to access the API. Also make a note of your API Key as you will need it for all requests to the API.
jQueryUI with autocomplete functionality
jQueryUI has an easy to use autocomplete tool that is great for allowing you to integrate our autocomplete endpoint to any text field on your website. In fact, it is the tool we are using throughout this website.
Here is how we recommend using it. First load the jQuery and jQueryUI libraries as well as our Air-port-codes library
<link rel="stylesheet" href="https://ajax.googleapis.com/ajax/libs/jqueryui/1.11.4/themes/smoothness/jquery-ui.css"> <script src="http://ajax.googleapis.com/ajax/libs/jquery/1/jquery.min.js" type="text/javascript"></script> <script src="https://ajax.googleapis.com/ajax/libs/jqueryui/1.11.4/jquery-ui.min.js" type="text/javascript"></script> <script src="air-port-codes-api-min.js"></script>
Now that everything is loaded, you can build a simple form with a textfield in it.
<form id="multi-form"> <input type="text" name="term" class="autocomplete" placeholder="City name or airport code" /> </form>
We can then initialize our apc object and setup our auto complete object that we pass in to jqueryUI's autocomplete plugin.
Make sure to add your API Key on line 5 and your API Key, if you need one on line 6.
<script> $(function() { $( '.autocomplete' ).each(function () { var apca = new apc('autocomplete', { key : 'xxxxxxxxxx', //secret : 'xxxxxxxxxxxxxxx', // Your API Secret Key: use this if you are not connecting from a web server limit : 7 }); var dataObj = { source: function( request, response ) { // make the request apca.request( request.term ); // this builds each line of the autocomplete itemObj = function (airport, isChild) { var label; if (isChild) { // format children labels label = '↳' + airport.iata + ' - ' + airport.name; } else { // format labels label = airport.city; if (airport.state.abbr) { label += ', ' + airport.state.abbr; } label += ', ' + airport.country.name; label += ' (' + airport.iata + ' - ' + airport.name + ')'; } return { label: label, value: airport.iata + ' - ' + airport.name, code: airport.iata }; }; // this deals with the successful response data apca.onSuccess = function (data) { var listAry = [], thisAirport; if (data.status) { // success for (var i = 0, len = data.airports.length; i < len; i++) { thisAirport = data.airports[i]; listAry.push(itemObj(thisAirport)); if (thisAirport.children) { for (var j = 0, jLen = thisAirport.children.length; j < jLen; j++) { listAry.push(itemObj(thisAirport.children[j], true)); } } } response(listAry); } else { // no results response(); } }; apca.onError = function (data) { response(); console.log(data.message); }; }, select: function( event, ui ) { // do something for click event console.log(ui.item.code); } } // this is necessary to allow html entities to display properly in the jqueryUI labels $(this).autocomplete(dataObj).data("ui-autocomplete")._renderItem = function( ul, item) { return $('<li></li>').data('item.autocomplete', item ).html( item.label ).appendTo( ul ); }; }); }); </script>
Need assistance?
If you need assistance getting our feed to work or simply have a suggestion, feel free to fill out our contact form.