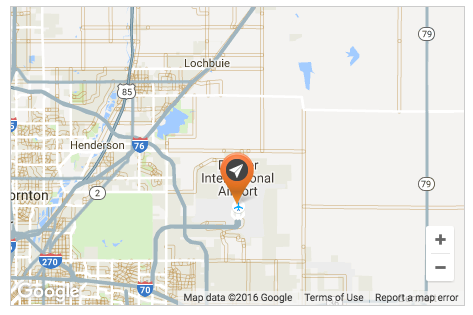
This request is great for retrieving all of the details about a given airport. It is very simple to use in that you simply need to pass in an airport code.
Download our example jQuery code, it comes with JavaScript and PHP libraries that make connecting to our API a breeze. Your API key can be found in your air-port-codes.com account and is necessary to be granted access privileges to any of our API's.
Use our interactive REST tool (Swagger) or our Postman collection to help you test the api and build your queries the right way. You'll need to login before you can use it with your API key.
Quick start
First login to your air-port-codes.com account and update your account settings by adding any domains that might need to access the API. Also make a note of your API Key as you will need it for all requests to the API.
Javascript
This request is useful for retrieving all of the data about a single airport. The only parameter necessary is the iata code of the airport. No jQuery or other frameworks are required to use our Air-port-codes library.
The basics
Here are the necessary parts to getting things working. First load our apc library that you downloaded earlier. Then initialize the api library, letting it know what api you want to access ('single' or 'single'). Initialize the apc object with your api key and any other necessary parameters. Setup your callbacks (onSuccess, onError). After that, you are ready to make your request with your search parameter.
<script src="air-port-codes-api-min.js"></script> <script> // initialize the apc library var apcs = new apc('single', {key : 'xxxxxxxxxx'}); // handle successful response apcs.onSuccess = function (data) { console.log(data); }; // handle response error apcs.onError = function (data) { console.log(data.message); }; // makes the request to get the airport data apcs.request('jfk'); </script>
PHP
If you use our PHP library, included in the download above, then it will be very easy to get a response back the Air-port-codes API. All of the curl functionality will be handled for you.
You start by including the apc class.
require_once('air-port-codes-api.php');
Next you create an array of parameters that will be used in your request to the API. Then pass in the parameters when you instantiate the apc class.
// Setup request parameters to override the configparams set in apc class $params = [ 'key' => 'xxxxxxxxxx', // this is required and can be found in your Air-port-codes account. // 'secret' => 'xxxxxxxxxxxxxxx' // the secret key, necessary for requests that don't provide a referrer ]; // 'multi' or 'single' type of request $apc = new apc('single', $params);
The final step is simply making the request. Just pass in the search term and your curl request will be automatically made to the Air-port-codes API.
$apcResponseObj = $apc->request('jfk');
You can now display the results however you want. The returned object will be a json decoded object.
<pre> <?= print_r($apcResponseObj); ?> </pre>
Need assistance?
If you need assistance getting our feed to work or simply have a suggestion, feel free to fill out our contact form.